Git is a version control system. If you want to save your code file at some stage it can be recovered in the future in required. Git is a way and Github is a platform. There are many other platforms like Bitbucket and all.
Install git
Check git –version
git config –global user.name
git config –global user.email
OR simply use git config –list
Go to the Project directory
git init, You will see hidden.git folder. The default branch is master.
git status
The best practice to check git idea online, click here.
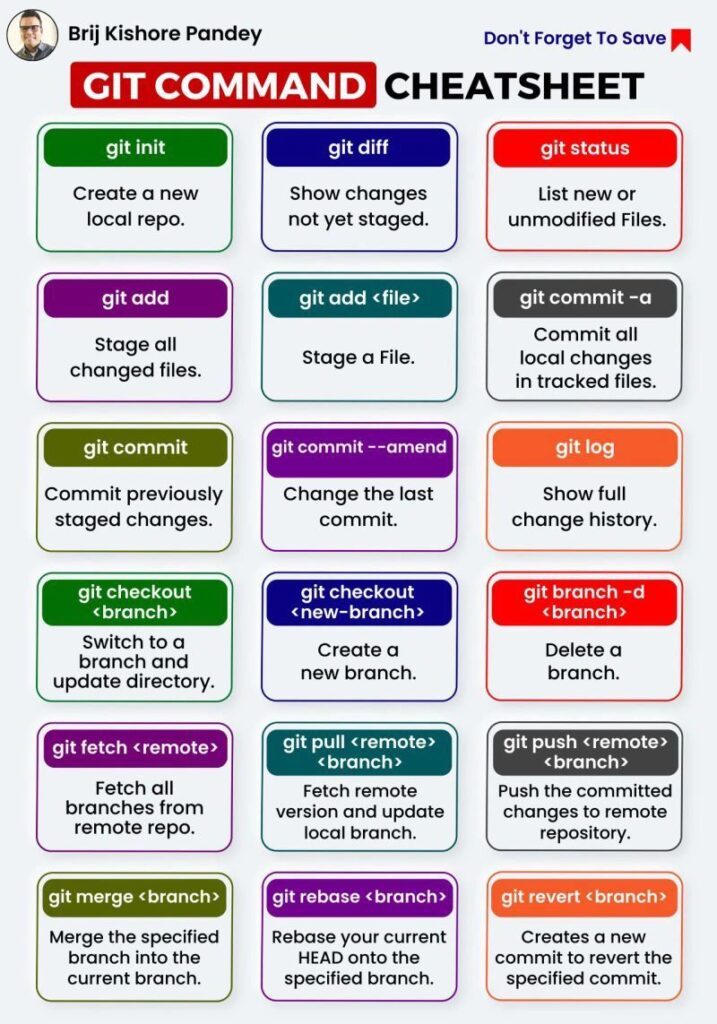
Branch concept: Generally our understanding is branch creates a separate code files. It creates a replica of code files. BUT IT IS NOT! A branch is just a way to comment/uncomment part of code in the same code file. When we write some code in the master branch and checkout to the staging branch, the code which we write in the master branch is going to be commented. Once we add new code to the staging branch and checkout to the master branch. The code which we wrote in the staging branch will be commented on.
And finally, when we merge the branches, all comments are removed and the code file is merging both codes of branches.
To display branches in a folder use: git branch
Change branch from master to staging by: git checkout staging
Create a new branch: git branch development
To create a new file: touch Introduction.txt
This file must be visible to that branch only. When you checkout to other branches, that file will hide from the display.
git add <filename> to track files in Version Control System or use git add . for all files
Commit concept: Commit is like a snapshot of the code file. We can restore back to that stage or even an earlier stage/commit. So better to commit the code when you are done with some section or segment of a part, for example, do it when login is done, when registration is done, or when you leave the file before handing it to someone else.
To add a markup on the file use git commit: git commit -m ‘Initial commit’
Now your local repository is ready. You need to push the local repo to the remote repository. For that, you have to create a new repo on Github either public or private. By creating a repo, you will see commands of http/ssh to push the local repo to remove the repo.
git@github.com:pdilip84/firstrepo.git
Now to push your local repo to remote repo, You have to authenticate first. As GitHub is not supporting password authentication anymore, You have to authenticate by SSH key. For that got to your GitHub account settings. You have to insert the SSH key which will authenticate you machine on GitHub.
How to generate a new SSH key in the local machine?
Just run this command in your terminal root folder: ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
This will create a new folder .ssh on your home/user-name folder. For me, it is home/dilip-parmar. Use ls -a to see all files with hidden files/directories. You will see a directory .ssh. Go there using cd .ssh.
Type ls to see files/directories under .ssh. You can see file id_rsa.pub there which is a public key. Another id_rsa is the private key. Never share that with anyone. Open that .pub file using the command: cat id_rsa.pub & you will see your public key like:
Copy that and paste it to the Github ssh key section and submit that. That’s it! EASY right? đŸ™‚
If you are setting up a new machine, you have to authenticate that machine on Github and for that you have to follow same procedure. After creating SSH successfully you have to run following commands into your local machine to tell that you are now using this global user & global email id.
git config --global user.name "Your name"
git config --global user.email youremail@email.com
This is a one-time procedure only. Once you are authenticated, You do not need to do this again for the same machine.
Now you can push your local repo to remote repo but for that, you have to define the remote repo path. You can check the existing path by git remote -v
To remove remote: git remote remove origin
Define remote path: git remote add origin git@github.com:pdilip84/firstrepo.git Where origin is the name of the repo. My repo name is firstrepo but we shortly called it origin.
Now push everything from the local repo to the remote repo(origin where the master is a branch)
git push -u origin master
some more concepts:
To merge bugfix branch to master: git merge bugfix
To better understand branch : git branch -a -v
To create new remote bugfix branch : git push -u origin bugfix
To remove local branch : git branch -d bugfix
To remote remote branch: git push origin –delete bugfix
OPEN THIS LINK TO UNDERSTAND COMMANDS IN A SINGLE IMAGE.
Now Pull & Fork:
When someone wants my repository to his/her GitHub account, he/she can do a fork (replicating everything to his/her account as a separate repository). He/she committed some changes and when he/she is done with better code, he/she can ask the main author of the repository to merge his/her changes. He/she can make a request owner by making a pull request.
The owner can review the code and can decide to merge. In this way, a simple repository exists many places as a separate repository and improve a lot and all those master minds make pull request to the owner, and the owner can improve the main repository with the help of masterminds!
Please note clone is a way to fetch the repository into the local host.
fork is to fetch it in your git account.
Check this image.

Here git clone and git pull looks same then what is the main difference between these 2 commands?
The answer is: Git clone copies all files to the local machine, while git pull only copies the modified files to the local machine. So if you have a remote repo in your local machine, just pull not clone.